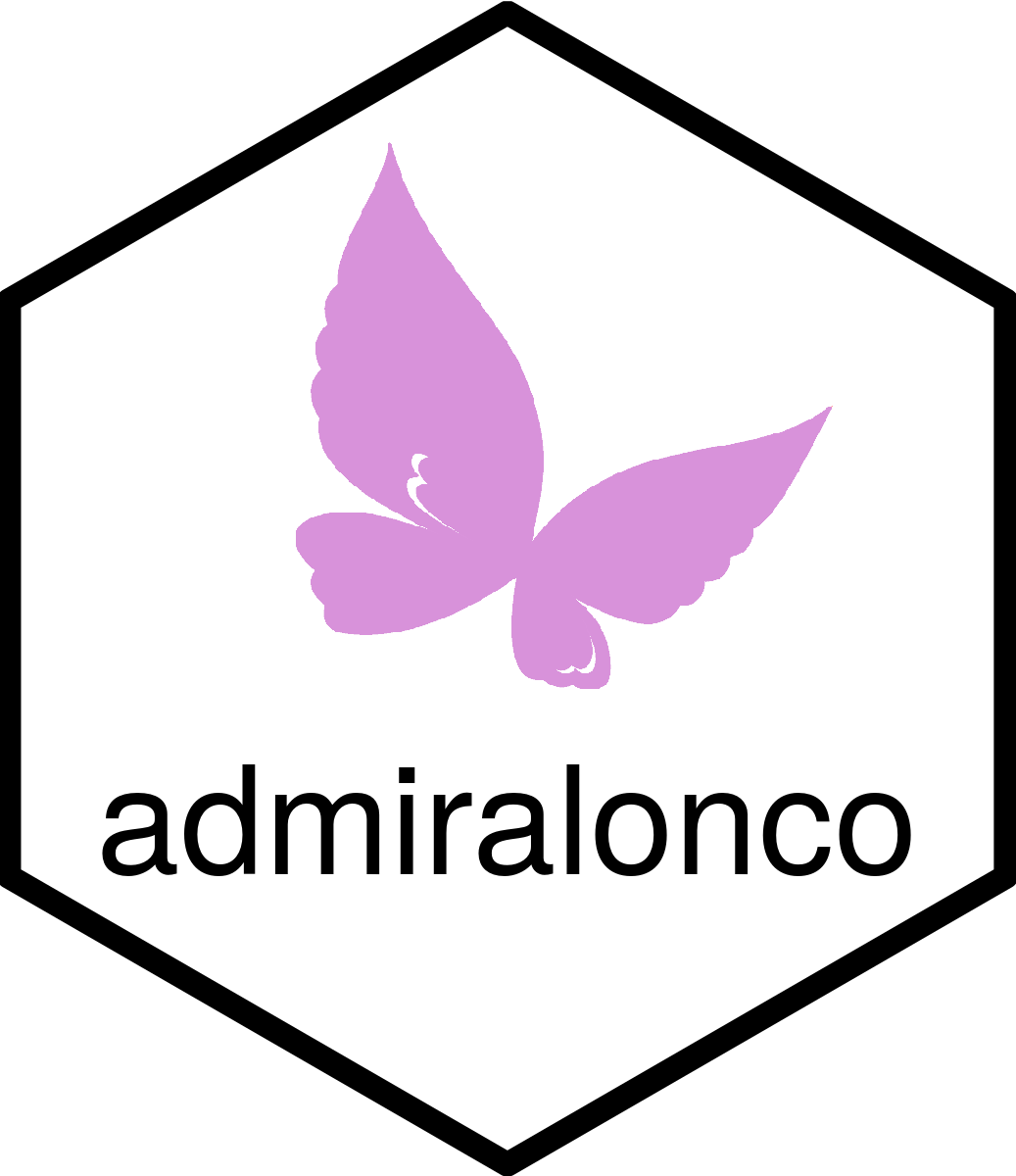
Adds a Parameter for the Last Disease Assessment
Source:R/derive_param_lasta.R
derive_param_lasta.Rd
Adds a parameter for the last disease assessment (optionally) up to first progressive disease
Arguments
- dataset
Input dataframe from which the Last Disease Assessment will be be derived from and added to.
The column
PARAMCD
and the columns specified insubject_keys
andorder
are expected.After applying
filter_source
and/orsource_pd
the columns specified bysubject_keys
andorder
must be a unique key of the dataframePermitted Values: a
data.frame()
object- filter_source
Filter to be applied to
dataset
to derive the Last Disease Assessment- order
Sort order, after which the last record shall be taken by the
subject_keys
to determine Last Disease Assessment. Created usingvars()
.Permitted Values: list of variables or
desc(<variable>)
function calls created byvars()
, e.g.,vars(ADT, desc(AVAL))
- source_pd
Date of first progressive disease (PD)
If the parameter is specified, the observations of the input
dataset
for deriving the new parameter are restricted to observations up to the specified date. Observations at the specified date are included. For subjects without first PD date all observations are take into account.Permitted Values: a
date_source
object (seedate_source()
for details)- source_datasets
Source dataframe to be used to calculate the first PD date
A named list of dataframes is expected (although for BOR) only one dataframe is needed. It links the
dataset_name
fromsource_pd
with an existing dataframe.For example if
source_pd = pd_date
withpd_date <- date_source( dataset_name = "adrs", date = ADT, filter = PARAMCD == PD )
and the actual response dataframe in the script is
myadrs
,source_datasets = list(adrs = myadrs)
should be specified.- subject_keys
Columns to uniquely identify a subject
A list of symbols created using
vars()
.- set_values_to
Columns to set
A named list returned by
vars()
defining the columns to be set for the new parameter, e.g.vars(PARAMCD = "LSTAC", PARAM = "Last Disease Assessment Censored at First PD by Investigator")
is expected. The values must be symbols, character strings, numeric values, orNA
.
Value
The dataframe passed in the dataset
argument with additional
columns and/or rows as set in the set_values_to
argument.
Details
Calculates the last disease assessment by accessing the last record
defined in subject_keys
after it has been arranged using the order
argument.
Creates a new parameter record (keeping all columns passed) from the last source
record (i.e. the last record defined in subject_keys
after it has been arranged
using the order
argument). One new record for each subject in the filtered
input dataset
is added to the input dataset
.
Records after PD can be removed using the source_pd
and source_datasets
arguments.
See also
ADRS Functions for adding Parameters:
derive_param_bor()
,
derive_param_clinbenefit()
,
derive_param_confirmed_bor()
,
derive_param_confirmed_resp()
,
derive_param_response()
Examples
library(dplyr)
library(lubridate)
library(admiral)
library(tibble)
library(magrittr)
adsl <- tribble(
~USUBJID, ~TRTSDT, ~EOSDT,
"01", ymd("2020-12-06"), ymd("2022-03-06"),
"02", ymd("2021-01-16"), ymd("2022-02-03"),
"03", ymd("2021-01-09"), ymd("2021-02-24"),
"04", ymd("2021-04-21"), ymd("2021-09-15")
) %>%
mutate(STUDYID = "a_study_id")
adrs <- tribble(
~USUBJID, ~PARAMCD, ~AVAL, ~AVALC, ~ASEQ, ~ADT, ~ANL01FL,
"01", "RSP", NA, "Y", 1, ymd("2021-04-08"), NA,
"02", "RSP", NA, "N", 1, ymd("2021-05-07"), NA,
"03", "RSP", NA, "N", 1, NA, NA,
"04", "RSP", NA, "N", 1, NA, NA,
"01", "PD", NA, "N", 1, NA, NA,
"02", "PD", NA, "Y", 1, ymd("2021-05-07"), NA,
"03", "PD", NA, "N", 1, NA, NA,
"04", "PD", NA, "N", 1, NA, NA,
"01", "OVR", 3, "SD", 1, ymd("2021-03-07"), "Y",
"01", "OVR", 2, "PR", 1, ymd("2021-04-08"), "Y",
"02", "OVR", 3, "SD", 1, ymd("2021-03-07"), "Y",
"02", "OVR", NA, NA, 1, ymd("2021-04-07"), NA,
"02", "OVR", 6, "PD", 1, ymd("2021-05-07"), "Y",
"03", "OVR", 3, "SD", 1, ymd("2021-01-30"), NA,
"03", "OVR", 3, "SD", 2, ymd("2021-01-30"), "Y",
"04", "OVR", NA, "NE", 1, ymd("2021-05-21"), "Y",
"04", "OVR", 5, "NON-PD", 1, ymd("2021-06-30"), "Y",
"04", "OVR", NA, "NE", 1, ymd("2021-07-24"), "Y",
"04", "OVR", NA, "ND", 1, ymd("2021-09-30"), "Y"
) %>%
mutate(STUDYID = "a_study_id")
pd <- date_source(
dataset_name = "adrs",
date = ADT,
filter = PARAMCD == "PD" & AVALC == "Y"
)
derive_param_lasta(
adrs,
filter_source = PARAMCD == "OVR" & ANL01FL == "Y",
source_pd = pd,
source_datasets = list(adrs = adrs),
set_values_to = vars(
PARAMCD = "LSTAC",
PARAM = "Last Disease Assessment Censored at First PD by Investigator",
PARCAT1 = "Tumor Response",
PARCAT2 = "Investigator",
PARCAT3 = "Recist 1.1",
ANL01FL = "Y"
)
) %>%
filter(PARAMCD == "LSTAC")
#> # A tibble: 4 x 12
#> USUBJID PARAMCD AVAL AVALC ASEQ ADT ANL01FL STUDYID PARAM PARCAT1
#> <chr> <chr> <dbl> <chr> <dbl> <date> <chr> <chr> <chr> <chr>
#> 1 01 LSTAC 2 PR 1 2021-04-08 Y a_stud… Last… Tumor …
#> 2 02 LSTAC 6 PD 1 2021-05-07 Y a_stud… Last… Tumor …
#> 3 03 LSTAC 3 SD 2 2021-01-30 Y a_stud… Last… Tumor …
#> 4 04 LSTAC NA ND 1 2021-09-30 Y a_stud… Last… Tumor …
#> # … with 2 more variables: PARCAT2 <chr>, PARCAT3 <chr>